A Complete Guide to Creating a Discord Chatbot

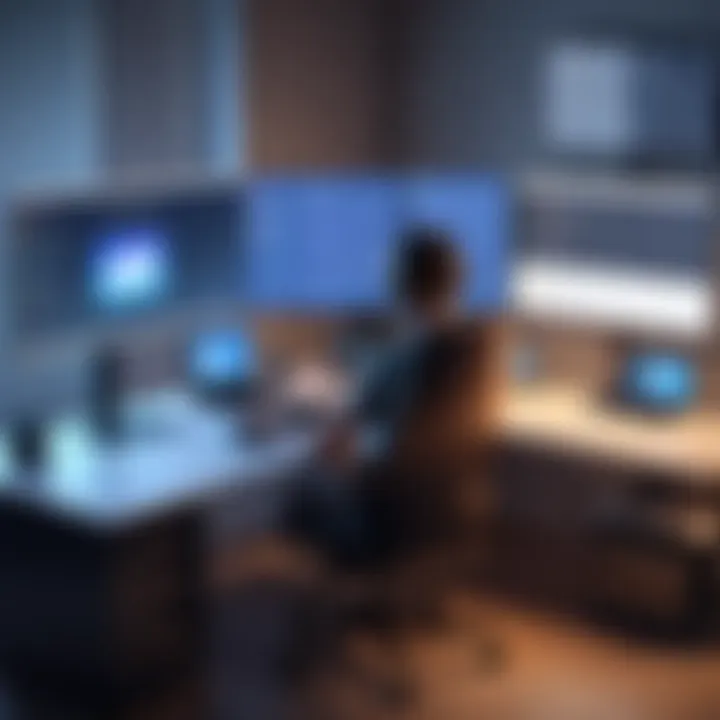
Intro
Building a Discord chatbot is not just a technical exercise; it's an opportunity to enhance engagement and foster community within your server. In the world of social platforms, Discord stands out for its versatility and robust features. Whether you're looking to create a bot that moderates conversations, provides information, or just adds some fun, understanding the key components and steps involved in the development process is paramount.
This guide will walk you through the essential elements of building a Discord chatbot. From the tools you will use to the programming languages that best suit your needs, every detail matters. If you’re new to coding or a seasoned developer, there’s something here for everyone. Prepare yourself for an engaging journey into the world of Discord development, where creativity meets functionality.
Key Features
Design and Build Quality
When you think about a bot’s design, you might not envision it like a physical product. However, the architecture of your Discord bot significantly affects its performance and usability. A well-structured codebase is foundational for maintaining efficiency and reducing bugs. Keep these aspects in mind:
- Modular Structure: Break down your code into manageable pieces. Each function should have one job. This makes it easier to debug and update.
- User Experience (UX): Create intuitive commands and responses. A bot should feel seamless to users, not something they struggle to understand or communicate with.
- Error Handling: Plan for the unexpected! Your bot should handle errors gracefully so that it doesn’t confuse or frustrate users.
Display and Performance
The performance of your bot is predominantly tied to how well it responds to user inputs and provides feedback. Here are a few considerations to keep in mind:
- Response Time: Users have little patience for lag. Optimizing your bot's response time can drastically improve user satisfaction.
- Scalability: Think ahead. If your community grows, will your bot handle increased traffic? Ensure its foundation is strong enough to support future expansions.
- Integration: Consider how well the bot integrates with Discord and other third-party services. A bot that works hand-in-hand with APIs opens up a world of possibilities for functionality.
Essential Tools and Languages
To get started with developing your Discord chatbot, you'll need a stack of tools. Here’s a summary of what’s useful:
- Node.js: Popular for bot development, it allows JavaScript to run outside the browser, making it ideal for building server-side apps.
- Discord.js: A powerful library for interacting with the Discord API using JavaScript. It simplifies code and makes implementation straightforward.
- Python: If you prefer Python, the discord.py library is a robust alternative that offers flexibility and ease of use.
Resources and Community
Besides the tools, tapping into available resources will save you headaches down the road. The Discord Developer Portal is an invaluable resource for documentation. Additionally, communities on platforms like Reddit can provide you with support and guidance from fellow developers. Engage with others, learn from their experiences, and don’t hesitate to ask questions.
"The art of programming is the art of managing complexity." — E. W. Dijkstra
By the end of this guide, you will not only have a technical understanding but also an insight into the creative possibilities that come with building a chatbot for Discord.
Preamble to Discord Bots
In the evolving landscape of online communication, Discord stands out as a pertinent platform. It caters to diverse communities—gamers, coders, book lovers, and more. But what elevates this service is its ability to integrate bots. These automated helpers can amplify user experiences and streamline interactions, making them essential in creating vibrant and engaged communities. This article will guide you through the nuts and bolts of Discord bots, ensuring you can harness their potential for your specific needs.
Understanding Discord as a Platform
Discord originated primarily as a chat platform for gamers. But over time, it has evolved into a multifaceted ecosystem, encompassing various community types. From hobbyist servers to professional networks, the capabilities offered by Discord are expansive.
Users engage through text channels, voice chat, and video calls. The design is intuitive, yet it provides enough depth to cater to power users. As a developer, understanding Discord's architecture is crucial. Each server can host multiple channels, leading to intricate layers of communication. This structure is what's at the heart of developing a Discord bot. You need to tap into these channels and understand how users interact with them.
Discord's API facilitates the connection between your bot and the platform. By learning how to navigate this API, you’ll gain the tools necessary to craft unique functionalities that resonate with your community. Moreover, hand-in-hand with this cognitive understanding, the practicality of deploying a bot is immensely forward-thinking in enhancing engagement.
Importance of Bots in Discord Communities
Bots serve as the backbone of engagement in Discord servers. They automate responses, manage user permissions, and often handle the tedious tasks that can take away from genuine interaction. Here are key reasons why bots are invaluable:
- Automation: Bots can manage operations like welcoming new members, moderating chats to remove spam, or even conducting polls, saving invaluable time for server admins.
- Customization: With tailored functionalities, bots can reflect the unique personality of a community, whether that's through fun commands, interactive games, or moderation tools.
- Enhanced Engagement: Bots can launch discussions, trigger games, and develop unique interactions that keep users coming back. An engaged community is a thriving one, after all.
- Data Management: They can help in tracking user behavior or preferences, allowing server owners to refine their strategies based on real insights.
As you delve into creating your Discord bot, remember its role is not just technical but also social. Your bot has the potential to shape how people interact within your space, bridging gaps and sparking conversations.
"A bot isn't merely code; it's a member of the community, sculpting the atmosphere and driving engagement."
Dominating the essence of Discord bots means embracing their ability to transform communication and foster a vibrant community spirit.
Prerequisites for Building a Discord Bot
When embarking on the journey of creating a Discord bot, understanding the prerequisites becomes indispensable. These foundational elements set the stage for a seamless development process and ensure that the bot you build is functional, efficient, and able to interact meaningfully with users. Without a grasp of these necessities, your bot may not meet its intended purpose or could fall short in performance.
Basic Programming Knowledge
To create a Discord bot, a certain base level of programming knowledge is essential. This doesn’t mean you need to be a coding wizard, but familiarity with programming concepts allows you to understand how to communicate with Discord’s servers, implement commands, and respond to user actions.
Common Languages Used
Most Discord bots are developed using programming languages like JavaScript and Python.
- JavaScript: Known for its versatility, JavaScript is commonly used with Node.js, allowing for a huge range of libraries and frameworks. This makes it a popular choice for many developers. Its asynchronous nature allows it to handle multiple connections smoothly, which is a plus for creating bots that talk to several users at once.
- Python: This language has gained traction due to its readability and simplicity. With the Discord.py library, it provides a straightforward way to build bots, making it a favorite among beginners who appreciate less code complexity.
Both languages come with their own set of strengths; JavaScript might feel more robust for larger applications, whereas Python may suit smaller, simpler bots better. Choosing the right language often boils down to your specific goals and existing knowledge.
Recommended Learning Resources
Diving into programming can be overwhelming, but there are numerous learning resources out there.
- Online Courses: Websites like Coursera and Udemy offer structured courses tailored to beginners. This helps in providing a guided approach, particularly for those unfamiliar with programming.
- Documentation and Tutorials: The official documentation for JavaScript and Python is often comprehensive. Using resources such as freeCodeCamp or Codecademy can boost your skills effectively.
- Forums and Community Support: Engaging with communities like Reddit or Discord servers dedicated to bot development can provide insights and real-time support from experienced developers.
These resources are excellent for starting your programming journey and help to solidify your understanding as you move into bot creation.
Familiarity with the Discord API
Understanding the Discord API is another critical prerequisite. The API is the backbone that allows your bot to interact with the Discord ecosystem. Basic knowledge of how the API works will significantly ease the development process.
API Documentation Overview
Navigating the Discord API documentation is vital for any bot developer. The API documentation explains how to make requests and what responses to expect.
- User-Friendly Format: The documentation is structured, making it easy to find relevant sections for different functions.
- Examples Provided: Code snippets and examples throughout the documentation can provide a clear reference point to understand how specific calls work.
Being acquainted with the documentation can save you a lot of time and frustration down the road as you’ll know where to find the information you need quickly.
Understanding API Rate Limits
One aspect that can trip up new developers is the API rate limits. Discord imposes limits on how many requests can be made over a set time to prevent abuse and ensure fair usage among all bots.
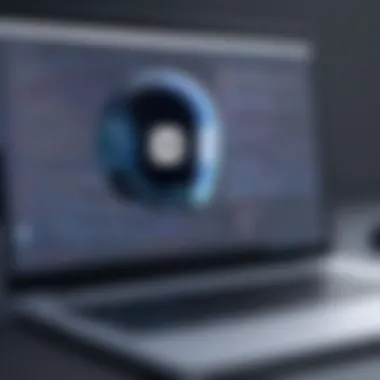
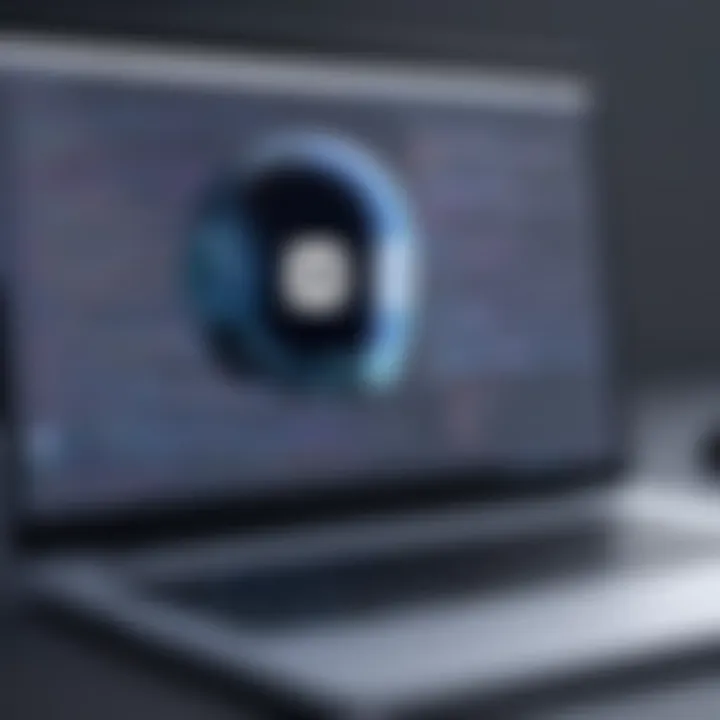
- Key Characteristic: Familiarity with these rate limits is essential. Every bot has capped usage, but understanding how to manage these limits can help avoid disruption in your bot's operation.
- Strategic Development: Knowing how many API calls you can make allows you to design your bot in a way that it doesn’t exceed these limits, creating smoother interactions.
Setting Up the Development Environment
Setting up the development environment is a crucial step in crafting your Discord bot. This phase establishes the foundation upon which you'll build your chatbot, ensuring that you have all necessary tools and frameworks at your disposal. An adequately configured environment can significantly enhance productivity and streamline the development process. It’s akin to a chef preparing his kitchen before cooking—having the right tools at hand makes a world of difference.
Choosing a Programming Language
Selecting the right programming language can be likened to choosing the right ingredients for a recipe. The language you elect to use will directly influence the simplicity, efficiency, and scalability of your bot.
JavaScript and Node.js
JavaScript, when paired with Node.js, emerges as a potent choice for bot development. One noteworthy aspect of JavaScript is its event-driven nature, which pairs well with the real-time requirements of Discord interactions. Node.js works seamlessly with JavaScript, allowing for non-blocking I/O. This means your bot can handle multiple requests without getting bogged down, an essential feature when dealing with the sometimes chaotic dynamics of a chat server.
One of the key characteristics of using JavaScript and Node.js is the extensive libraries available, such as Discord.js, which simplify the API interactions. The ease of integration is a unique feature, making it straightforward to manage Discord functionalities like sending messages or responding to user commands. The downside? If you’re not already familiar with JavaScript, the learning curve can be a bit steep, especially if you venture into asynchronous programming.
Python and Discord.py
On the other hand, Python, coupled with the Discord.py library, offers a different flavor for bot development. Python’s syntax is often praised for being clean and readable, making it a popular choice among newcomers. The Discord.py library provides a lot of out-of-the-box functionality that simplifies bot interactions, which can be immensely appealing for fresh developers.
A critical feature of Python in this context is its vast ecosystem—there’s a library for nearly everything. This adaptability allows developers to extend the bot's capabilities easily, whether that be for data processing or integrating machine learning algorithms. However, Python may lag behind Node.js when it comes to handling high concurrency, though for many simple bots, this is a non-issue.
Installing Necessary Tools
Once you’ve chosen your programming language, the next step involves installing the necessary tools. This stage is akin to gathering your kitchen gadgets; each tool plays a specific role in making your development smoother.
Code Editors
A good code editor is an indispensable tool for any programmer. Editors like Visual Studio Code or Atom stand out for their rich features such as syntax highlighting, debugging support, and plugin availability. These characteristics make development more efficient and enjoyable. Visual Studio Code, in particular, offers a user-friendly interface and integrates nicely with version control systems like Git. On the flip side, some might find that the sheer number of customization options can be overwhelming at first.
Package Managers
Package managers such as npm for JavaScript or pip for Python facilitate the installation and management of libraries. They allow developers to pull in external libraries easily, unavoidable in the ever-evolving landscape of programming. They save time that would otherwise be spent manually configuring dependencies, making them a beneficial addition to your toolkit. However, a potential downside lies in versioning issues; libraries can sometimes become incompatible with others or lose support. Keeping track of these versions is crucial to avoid headaches down the road.
"A well-configured development environment paves the way for successful bot deployment and ongoing maintenance."
In summary, setting up the development environment is not just about having the right tools; it's about creating a conducive atmosphere for your development journey. Choosing the correct programming language and ensuring you have effective tools can play a pivotal role in the quality and success of your finished Discord bot.
Creating Your Bot on Discord
Creating a Discord bot is the keystone of enhancing interactions within the platform. It’s not just about adding some lines of code and calling it a day. It involves a plethora of decisions that can shape how users experience your bot. Every detail, from functionality to user engagement, counts.
Bots serve various purposes, like moderating discussions, sending alerts, or even managing game statistics. Thus, pinpointing how your bot will operate is fundamental. Moreover, aligning its functionalities with the needs of your server impacts not only how users perceive your bot but also how they integrate it into their daily use.
Setting Up a Discord Account
Before diving deep into bot development, ensuring you have a Discord account is crucial. Your account serves as the foundation of your bot's identity within the discord ecosystem. When you sign-up, you can access all the necessary features to manage and develop your bot.
- Create an Account: If you don’t have one, it’s as simple as visiting the Discord website or downloading the application and following the sign-up prompts.
- Verify Your Account: Sometimes you might need to verify your email. This isn’t just for security but is beneficial in gaining full access to bot features.
- Join/Create a Server: Engaging with your bot in a controlled environment, such as a personal server, allows you to test functionalities without interference.
Registering Your Bot with Discord
Once your account is good to go, the next step involves registering your bot. This process is where your bot gets its online identity – think of it as putting a name tag on your creation.
Creating a New Application
Creating a new application in Discord is akin to laying the groundwork for your bot's functionalities. This step is vital because it links your account with the bot, allowing you to define its capabilities.
- User-Friendly Interface: The application page is straightforward; with just a few clicks, you start defining your bot’s identity.
- Distinct Identity: You can customize various settings, including your bot's profile picture and name, making it recognizable to users.
Since Discord has a robust structure, developers find Creating a New Application a beneficial step. Meanwhile, some might overlook it, thinking it is less significant compared to actual coding. However, if not done correctly, your bot might miss functionalities that could engage users more actively.
"A bot without a sound identity is like a voice lost in a crowded room."
Generating a Bot Token
Generating a bot token is akin to issuing a birth certificate for your bot. This token is unique and serves as the key to interact with the Discord API. Failing to safeguard it appropriately could lead to breaches where unauthorized individuals can manipulate your bot.
- Access Control: This token is needed to authenticate your bot's presence within servers and ensures it can execute commands.
- Keep it Secret: The token must be kept confidential. If shared, it can grant others the ability to control your bot entirely, which could lead to adverse effects.
The unique characteristic of Generating a Bot Token is its critical role in establishing secure communication between your bot and Discord’s servers. By following best practices in managing these tokens, including regenerating them regularly, developers can safeguard their applications against unwanted exploits.
In essence, creating your bot involves stepping through organized processes that build a coherent structure. Each component lays the groundwork for the functionalities that will soon follow. From setting up your Discord account to the careful steps of registration, these actions form an essential foundation. Understanding the importance of each step equips you with better insights into successful bot deployment.
Bot Development Basics
Understanding the fundamentals of bot development is crucial for anyone venturing into the world of Discord chatbots. This section acts as the backbone of your journey. By grasping the core principles, you set the stage for more complex functionalities.
Establishing a Simple Bot
Creating a basic bot is like laying the first stone of a grand structure. It may seem simplistic, yet it provides the necessary foundation upon which you can build additional features and capabilities. With a solid start, you gain the confidence to delve deeper into bot development.
Connecting to Discord
Connecting your bot to Discord is the first and foremost step in this process. It involves establishing a link between your bot code and Discord's servers, enabling your bot to interact with channels and users seamlessly. One key aspect of this connection is the Discord API, which serves as the bridge.
The allure of connecting to Discord lies in its simplicity. Developers predominantly use libraries like Discord.js (for JavaScript) or Discord.py (for Python), making it a widely favored method among tech enthusiasts and novices alike.
A unique feature of this connection is the bot token. This is a kind of ID that lets your bot authenticate with Discord and act within servers. Safeguarding this token is paramount. If it gets into the hands of an ill-intentioned user, they can control your bot.
Overall, connecting to Discord is an essential step that sets the groundwork for your bot's functional capabilities, while allowing you to explore further development opportunities later on.
Basic Commands Implementation
Once connected, the next logical step is implementing basic commands. This is akin to teaching your bot a few key phrases – it can greet users, respond to queries, and perform basic functions. The beauty of this stage is in its adaptability. You can craft commands according to your community's needs.
The command framework is the cornerstone of user interaction. It allows your bot to recognize inputs and react accordingly. Along with connecting to Discord, it makes your bot more engaging and functional.
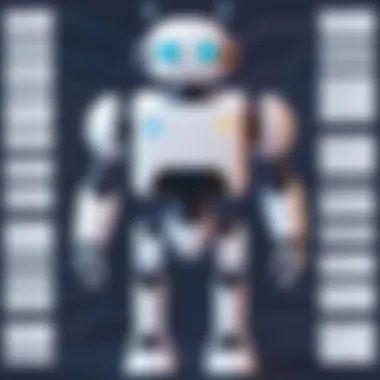
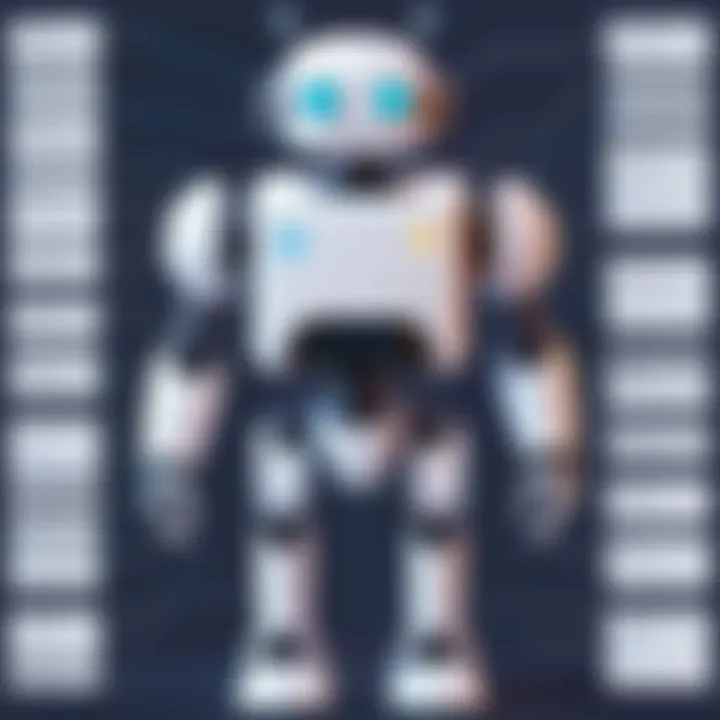
A notable feature here is ease of modification. As your bot evolves, you can add, remove, or alter commands with relative ease. This flexibility is particularly valuable in a rapidly changing digital landscape where user expectations shift often.
However, one must keep in mind that while starting with basic commands is beneficial, it’s easy to overlook documentation. Thoroughly understanding how commands work and how they can be structured will serve you well as you scale your bot's complexity.
Handling User Interactions
Handling user interactions is where the real magic happens. It's about creating a responsive and engaging experience for users. After establishing a basic bot, this step is vital for keeping users interested and active.
Responding to Messages
The ability of your bot to respond to messages effectively is crucial. It's the bridge between automation and user relationships. By effectively processing user inputs, your bot can provide timely and accurate responses, which keeps the interaction lively.
One of the key characteristics of responding to messages is that it fosters community engagement. When users receive immediate feedback, they feel valued and more likely to participate in discussions.
A strong aspect of this functionality is personal customization – you can tailor responses to fit the tone and needs of your community. This feature makes responding to messages an art as much as it is a function.
Creating Command Prefixes
Another notable aspect is the creation of command prefixes. Command prefixes let users know when they are issuing a command versus just chatting. This means your bot won’t get flooded with messages that should not trigger a command.
By default, many developers opt for a simple prefix like “!” or “.”, which becomes intuitive for users over time. This little nuance — despite its simplicity — has significant implications for smoothing out interactions.
Over time, you can evolve these prefixes to include context-specific variants, enhancing user-friendliness even more. However, with unique prefixes comes the need for clarity. If prefixes are overly complex, they can lead to confusion among users. It's all about striking that right balance.
In the bot development world, the little details — like connecting properly or creating effective command prefixes — can turn an ordinary bot into a beloved community member.
By mastering the basic elements of bot development, you create a strong foundation that enables growth, user engagement, and, ultimately, a successful chatbot. This basic knowledge becomes indispensable as you venture into advanced features in the later sections.
Implementing Advanced Features
Adding advanced features to your Discord bot can make it more versatile and engaging. By leveraging these capabilities, you're not only enhancing its functionality but also improving user experience. This section dissects two major areas: integrating APIs for external resources and adding multi-server support.
Integrating APIs for Enhanced Functionality
Integrating APIs can elevate your bot’s game significantly. With access to vast amounts of data and functionalities from external sources, your bot becomes far more useful. Many developers see this aspect as the bread and butter of advanced bot features because it allows for a tailored experience for users.
Fetching Data from External Sources
Fetching data from external sources can be a game changer. Imagine your bot is not just a collection of commands but a live data feed. For instance, pulling real-time weather reports or news can keep your server buzzing with fresh information. It's an attractive feature, especially for community servers.
The key characteristic of this is its ability to keep users informed without them having to leave Discord. It's beneficial because it centralizes information and encourages user interaction, making your bot an integral part of the community. However, you must bear in mind that it's crucial to manage the rate limits enforced by these APIs. A bot that makes too many requests can quickly run into a wall, causing headaches for both developers and users.
A unique aspect is the versatility of data types you can fetch. Whether you're looking for a simple text output or more complex visual data like graphs, there are APIs for that. Advantages include a wealth of useful data at your fingertips, while disadvantages can entail the need for consistent updates if API endpoints change or become obsolete.
Using APIs for Game Stats
Game stats APIs are particularly popular among gaming communities. They can track player scores, stats, and even match histories. Integrating such APIs can make your bot a go-to resource for gamers wanting the latest updates or to analyze their performance.
The primary benefit of this feature is its engagement factor. Gamers love to geek out over stats; thus, having that data presented directly in Discord keeps them coming back. The unique capability here is that these APIs often provide real-time updates, making the data fresh and relevant.
However, be mindful that not every game offers a stable API. Changes or outages can affect the availability of your bot's features. The upside? When everything is running smoothly, your bot can significantly enhance player engagement and community dynamics, making it a valued asset.
Adding Multi-Server Support
As your Discord bot grows in functionality, so should its capabilities to serve multiple servers. Multi-server support makes your bot scalable. It cannot be understated how vital this is if you aim to reach a wider audience.
Scaling Your Bot's Capabilities
Scaling your bot means it can handle increased user counts and interactions efficiently. The importance of this lies in the potential for growth; as your bot becomes popular, it will attract a larger user base.
One key characteristic of scaling is utilizing efficient code and architecture. Adopting best practices can save time and energy as you expand. A unique feature of scaling is the use of load balancing; distributing user requests to optimize performance. Advantages include smoother operation under high traffic, while disadvantages may include increased complexity in the codebase.
Data Storage Solutions
Data storage solutions are crucial for multi-server operations. You will need a solid method for storing user data, settings, and specific configurations for each server. The great thing here is that there are various choices from traditional databases like MySQL to NoSQL options such as MongoDB.
The key feature of data storage solutions is their ability to retrieve and store data efficiently. This not only ensures your bot runs smoothly but also maintains user experience across different servers. The benefit is a tailored experience for each server, while the drawback might be the learning curve involved in setting up and managing these databases.
In summary, implementing advanced features like API integrations and multi-server support elevates your Discord bot from basic to complex. But balancing functionality with user needs is essential for long-term engagement.
Testing and Debugging Your Bot
Testing and debugging are crucial aspects when it comes to developing a Discord bot. Think of it as the final polish you'd give to a diamond before showcasing it to the world. Here, both developers and users can truly reap the rewards of a well-tested bot. Reliable performance means better interaction for users and an overall smoother experience. As your bot interacts with countless users, it becomes imperative to ensure it can handle all sorts of scenarios. A well-tested bot not only enhances user satisfaction but also reduces long-term maintenance costs.
Identifying issues before they reach your user base is easier when a thorough testing strategy is employed. It's essential to note the balance between adding new features and ensuring existing functionalities are working seamlessly. This balance is what keeps your bot not just alive, but thriving in the dynamic world of Discord.
Common Issues and Troubleshooting
Even the best-laid plans can go awry, and developing a Discord bot is no different. Users may encounter a variety of issues ranging from incorrect command responses, broken integrations, or even login errors. Recognizing these common issues early on is half the battle won.
Some common hitches can include:
- Permissions problems: Bots need specific permissions to operate correctly. Misconfiguration can lead to limited functionality.
- Rate limits: As discussed earlier, the Discord API has restrictions on how often you can make requests. Ignoring these limitations can lead to your bot being blocked temporarily.
- Webhook failures: Sometimes, your bot's communication with external APIs might fail. Monitoring these interactions can unveil unseen problems.
- Unexpected crashes: Clearly, nobody likes a bot that goes offline without a word. Logs will help figure out when and why these occurrences happen.
To troubleshoot effectively, be relentless in looking for logs and feedback that can help pinpoint exactly where the issue resides. Changes to the code must be tested, with every adjustment leading you closer to a more stable product.
Best Practices for Testing Bots
When it comes to testing Discord bots, having a structured approach can make all the difference. Let’s dive into a couple of best practices that could make testing more efficient.
Unit Testing Frameworks
Unit testing frameworks provide developers with the tools necessary to automate testing of individual units of code. This approach ensures that small bits of functionality—things like commands and responses—work as expected. A notable characteristic is how unit tests can be run independently, making it clear where any issues lie.
It's a beneficial choice because you can catch bugs early on, preventing a cascade of errors that might occur later in development. A popular framework for bot development in JavaScript is Mocha; while in Python, you might consider unittest. The unique feature of these frameworks is the ease with which you can set them up to run automatically upon code changes.
However, relying solely on unit tests might limit your testing scope. They are great for checking isolated units but can miss flaws when components interact.
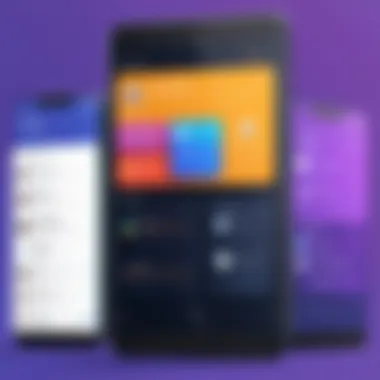
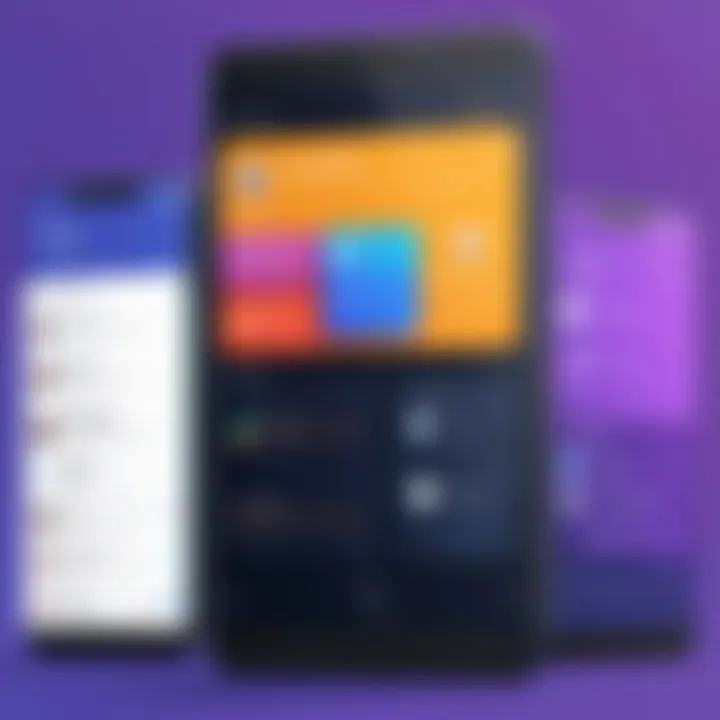
User Feedback Loops
User feedback loops involve gathering feedback from users after they interact with your bot. This aspect is essential because real-world usage often exposes problems that developers simply don't see during testing. One key characteristic of user feedback loops is their iterative nature. Each piece of feedback leads to insights and adjustments, gradually improving the bot.
Using platforms like Reddit and social media channels where your bot operates can be an effective way to gather opinions. A unique feature is how this feedback can drive priorities in development; if users frequently report issues or request features, it provides a clear direction for your next steps.
While incorporating user feedback is invaluable, you must manage it carefully. Not all feedback will be constructive or easy to implement, and you can’t please everyone. Thus, filtering through this data is a critical skill in maintaining your bot's effectiveness.
"Testing is not just a phase, it's a crucial pillar in building a successful bot."
Deployment and Hosting Options
When you’ve crafted a Discord bot, the journey isn’t over yet. Deployment and hosting options play a critical role in ensuring your bot runs smoothly and efficiently. You might have the best code in the world, but if it’s not housed properly, you could run into all sorts of hiccups down the line. Whether you choose to host your bot in the cloud or set it up on your own server, understanding these options can save you a lot of headaches.
Cloud Hosting Solutions
Cloud hosting has become a popular choice among developers because of its flexibility and scalability. When your bot gains traction and starts to accumulate users, you’ll want hosting options that can adapt to increased demand without breaking a sweat.
Choosing the Right Provider
Selecting a cloud hosting provider requires careful thought. Not all services are created equal; some stand out due to their excellent uptime, customer support, and tailored offerings for developers. For instance, services such as Amazon Web Services (AWS) or Google Cloud Platform offer scalable resources suited for bot hosting needs. The key characteristic of these providers is their infrastructure that can easily adjust as your bot's user base grows.
- Scalability: As user numbers increase, being able to scale resources is vital.
- Global reach: Many services provide data centers around the world, which improves connection times for users.
However, such advanced features can come at a price that might not fit into everyone's budget. This brings us to consider the cost-benefit balance.
Cost-Benefit Analysis
Engaging in a cost-benefit analysis helps you determine whether cloud hosting is financially viable for your Discord bot. The primary appeal here is the potential for long-term savings. Many cloud providers offer a pay-as-you-go model that lets you pay only for the resources you use.
- Affordability: This can be especially beneficial for small developers or hobbyists.
- Maintenance: With cloud solutions, you often don’t have to worry about upkeep, as that responsibility falls on the provider.
That said, there's a noticeable dependency on the provider’s availability. Downtime at their end means downtime for your bot too, which isn't an ideal situation for your users. This dependence is something to ponder deeply before jumping in.
Self-Hosting Considerations
On the other end of the spectrum, self-hosting offers more control but comes with its own set of challenges. This route is often chosen by those who value autonomy over convenience.
Technical Requirements
Before self-hosting your Discord bot, it’s crucial to know the technical requirements involved. A solid understanding of server management, networking, and the bot’s specific tech stack is essential for a successful deployment. This can range from having the right server specs to running the necessary software applications.
- Hardware: You’ll need a server that can handle both the bot's needs and the expected user load.
- Software: Ensure you have the correct operating system and libraries installed that support your bot’s coding language.
While self-hosting grants you freedom, the caveat is that it often requires a greater time investment, in both setup and ongoing maintenance.
Security Concerns
With great power comes great responsibility, especially regarding security. Self-hosting means you must secure your server against both malice and data breaches. It’s not just about keeping the bot running; it also means safeguarding user data and privacy.
- Firewalls: Setting up strong firewalls is crucial to protect your server from external attacks.
- Regular updates: Keeping your software and dependencies updated helps mitigate vulnerabilities.
The flexibility of self-hosting may come with risks that can be challenging for those unfamiliar with cybersecurity. Ensuring robust security protocols is a must if you opt this route.
In summary, whether you decide on cloud hosting solutions or self-hosting, each has its merits and pitfalls. Understanding what you need from your hosting provider or self-hosted setup can make the difference between a bot that works seamlessly and one that struggles to stay online.
Maintaining and Updating Your Discord Bot
When you’ve managed to build a robust Discord bot, one might think the work is done. Contrary to that belief, maintaining and updating your Discord bot is just as crucial as the initial development phase. Over time, the landscape of technology evolves—new APIs arise, Discord updates its features, and user's expectations shift. Regular updates not only enhance the functionality of your bot but also ensure it stays aligned with the community's needs.
Monitoring Performance
To make sure your bot runs smoothly and effectively, you need to keep an eye on its performance. This involves regularly analyzing its behavior, understanding how it interacts with users, and pinpointing any areas that could use improvement.
Utilizing Logging Tools
Logging tools are indispensable when it comes to performance monitoring. They serve as the eyes and ears for your bot, capturing activities and errors that occur during its operation. One significant characteristic of logging tools is their ability to track events and exceptions, which can be invaluable for debugging issues quickly. For example, with tools like Winston or Morgan in Node.js, you can log everything from info messages to critical error events.
Through the extensive records generated, you gain better insight into how your bot performs under different conditions. However, there might be a disadvantage as well: excessive logging can clutter your server space or slow down performance if not managed well.
Identifying Usage Patterns
Identifying usage patterns is another critical aspect of monitoring performance. By analyzing how users interact with the bot, you can unearth trends and behaviors that help you understand what's working and what's not. The key here lies in studying metrics like the frequency of commands used and the number of simultaneous users engaging with your bot. Tools like Google Analytics can assist in gathering this data.
A unique feature of recognizing usage patterns is that it allows you to tailor updates specifically to your user base's interests. On the flip side, it can sometimes lead to over-generalization; if you solely base your decisions on what's popular, you could overlook niche features valued by some users. Thus, balance is essential.
Implementing User Feedback
User feedback is like gold dust for maintaining your Discord bot. Engaging with your bot's users allows you to gather insights directly from the source—your audience. This not only demonstrates that you value their opinion but also helps you make informed decisions about future updates.
Developing surveys or feedback forms gives users an outlet to voice their thoughts. Equally, keeping an eye on discussions in your server channels or on platforms like Reddit can provide qualitative data that informs your development choices. The key issue here is being proactive; waiting for users to offer feedback might lead to missed opportunities for improvement. A responsive bot that evolves with its community will certainly stand out in the crowded bot marketplace.
End
In wrapping up our exploration into the world of Discord chatbot development, it’s crucial to highlight the significance of this journey. Building a Discord bot isn’t just about writing a few lines of code; it’s about creating a dynamic tool that can foster and enhance community engagement. As we sifted through languages, APIs, and deployment logistics, it became apparent that each of these elements plays a distinct role. The intersection of technology and community-building is where the true value of a Discord bot lies.
The benefits of understanding these concepts cannot be overstated. It empowers developers to unleash their creativity, allowing them to build bots tailored to their community’s needs. With the right skills and knowledge at hand, anyone could transform a mere idea into a living, breathing bot that interacts with users, automates tasks, and brings a lifeline to discussions.
As this guide has shown, one must consider not only the technical requirements but also the community’s preferences. In the vast universe of Discord, a well-maintained bot that responds to the desires of its users can be a beacon of engagement. Regular updates and user feedback loops ensure that your bot remains relevant and efficient over time. The care you put into your bot now will pay dividends in user satisfaction later.
"A well-crafted Discord bot is like a good conversation partner; it understands, engages, and brings joy to the community."
Ultimately, the continual development of bots in the Discord landscape speaks to the heart of its community. It’s an ongoing process, one that invites enthusiastic developers to keep learning and evolving.
Recap of Key Takeaways
- Building a bot is multifaceted: It involves choosing programming languages, understanding the Discord API, and setting up a suitable environment.
- Community matters: Listening to the users is key to maintaining relevance.
- Regular updates enhance performance and user satisfaction.
- Technical skills alone aren't enough: Engaging with community dynamics is vital.
Encouragement for Further Development
As we conclude this guide, consider this an invitation to delve deeper into the vast resources available online. The world of Discord is ever-evolving, and so should your skills and knowledge. Keep an eye on the latest trends and advancements in APIs, programming languages, and community preferences. Don’t shy away from experimenting with new features and functionalities.
Engage with online communities, read relevant articles, and participate in forums on platforms like Reddit or GitHub. Every interaction can yield new ideas and inspirations. The only limit to what your bot can achieve is your imagination and willingness to learn.
Ultimately, being proactive in your learning journey and continuously refining your skills will not only benefit you as a developer but also enrich the communities you serve. Embrace the challenges ahead, and happy coding!