Creating a Discord Bot: A Comprehensive Guide
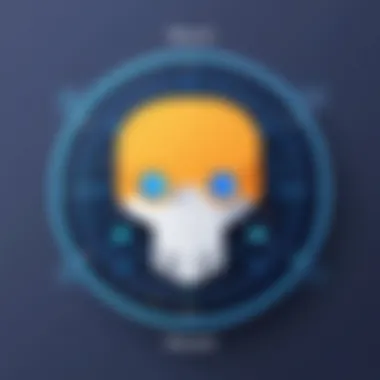
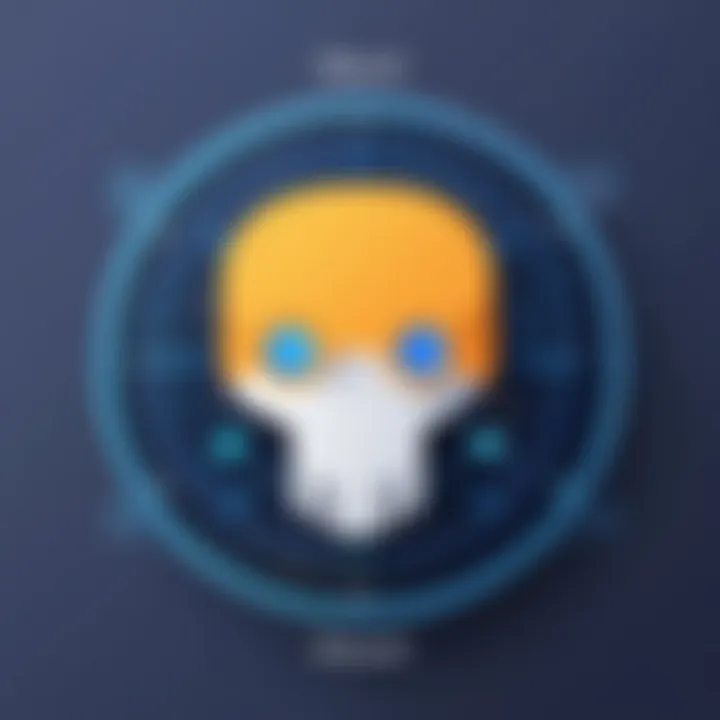
Intro
Creating a bot for Discord offers users a unique opportunity to enhance their communication and community engagement within the platform. Discord has gained immense popularity, especially among gamers, tech enthusiasts, and various interest groups. A bot can automate tasks, provide information, or even entertain. It can greatly improve the user experience by reducing the manual workload for administrators and allowing for a more organized and interactive environment.
This guide is tailored for individuals who may have varied experience with programming, from novices who are just dabbling in code to those who possess a solid understanding of programming concepts. The intent is to demystify the process of creating a Discord bot and to make it accessible and comprehensible while ensuring that each step along the way is thoroughly covered.
Key Features
The significance of a well-designed bot can be seen in several key features:
- Functionality: A bot should accomplish specific tasks effectively, whether it’s moderating chats or providing updates.
- Ease of Use: The bot interface should be user-friendly, allowing users easy access to its features.
- Customization: Flexibility in modifying features according to user preferences is vital. Users should be able to configure settings without extensive technical knowledge.
- Performance: The bot should operate efficiently, handling commands and tasks promptly, even under heavy load.
Understanding the Discord API
Before diving into bot creation, it is crucial to comprehend how the Discord API operates. The API acts as a bridge between your code and Discord, enabling interaction with the platform’s features. To understand this better, read the documentation available on the Discord Developer Portal.
Here are some fundamental elements to focus on:
- Authorization: Acquiring a bot token is essential for your bot to interact with Discord services.
- Endpoints: Learn about the different API endpoints that you will be using to send or receive messages.
"The Discord API provides a powerful way to interact with the Discord platform and build features that enhance user experience."
Setting Up Your Development Environment
To begin, set up a suitable development environment. Here’s what you typically require:
- Node.js: Download and install Node.js to run your bot.
- Code Editor: Use Visual Studio Code or any editor you prefer.
- Discord.js Library: This library simplifies the interaction with Discord’s API. Install it using npm:
Coding Fundamentals
Creating a basic bot starts with writing some code. It's beneficial to start simple. Here is a minimal code snippet that demonstrates how to create a basic bot that responds to messages:
This code sets up a bot that replies with "Pong!" whenever a user sends the command "!ping". Replace with your actual bot token.
Best Practices for Bot Management
- Regular Updates: Keep your bot updated to ensure compatibility with Discord changes.
- Error Handling: Implement proper error handling to prevent crashes and provide clear feedback on failures.
- Security: Guard your bot's token and any sensitive information carefully.
The End
As evident, creating a Discord bot involves understanding both technical and community aspects. Each step is significant, from setting up your environment to ensuring your bot operates effectively. With the proper knowledge and tools, anyone can create a bot that enriches the Discord experience. This guide serves as a foundation for developing, deploying, and managing your bot effectively.
Preamble to Discord Bots
In recent years, Discord has emerged as a powerful platform for community engagement and communication. The ability to create and deploy bots enhances the user experience by automating tasks, managing server activities, and providing interactive features. Understanding the concept of Discord bots is vital for both novice and experienced developers alike. This knowledge not only aids in leveraging Discord’s full potential, but also broadens the horizon for creating innovative applications.
Understanding Discord API
The Discord API serves as the backbone for developing Discord bots. It is a set of rules and protocols that allow developers to interact programmatically with Discord's features. By using the API, bots can send and receive messages, manage server roles, and engage users in various ways.
Accessing the Discord API requires a registered application through the Discord Developer Portal. This registration process generates APIs keys and tokens, which authenticate the bot and establish connectivity with Discord's servers. Working with the API involves understanding WebSocket connections, HTTP requests, and JSON data structures, which are crucial for effective bot functionality. Thus, familiarity with the API is an essential step for any developer wanting to create a bot.
Purpose and Functionality of Bots
Discord bots serve a myriad of purposes across countless servers. The range of functionalities can vary significantly, from simple moderation tasks to complex game integrations. Here are some primary functions of Discord bots:
- Moderation: Bots can automate moderation tasks, such as filtering messages, kicking or banning users, and managing roles. This reduces the burden on server admins, ensuring a smoother community experience.
- Utility: Many bots provide essential utilities like reminders, polls, or FAQs, streamlining information dissemination within communities.
- Entertainment: Bots can host games, trivia, or music playback, enriching the engagement of users on the server.
The essence of bots lies in enhancing user interaction and community management. Understanding their purpose is crucial for developers aiming to create successful bots that resonate well with users. The importance of this section cannot be overstated; it sets the groundwork for deeper explorations in bot development. Adoption of best practices and awareness of user needs guided by the functionalities described will help in creating a more impactful bot.
"Bots can be the bridge between users and the functionalities of a Discord server, optimizing communication and interaction."
Setting Up Your Development Environment
Setting up your development environment is a critical first step in the journey of creating a Discord bot. It lays the groundwork for building, testing, and deploying the bot effectively. A well-configured environment not only enables smooth coding but also enhances productivity by reducing errors and frustration during development. This section focuses on the essential aspects of choosing programming languages and installing necessary software that will streamline the development process.
Choosing the Right Programming Language
Selecting a suitable programming language is essential for the successful creation of a Discord bot. Each language has its strengths and weaknesses that can affect the bot’s performance, ease of use, and maintenance. Below are three popular languages you can consider:
JavaScript
JavaScript is the most widely used language for developing Discord bots, particularly due to its compatibility with the Node.js runtime. Its event-driven architecture suits the real-time nature of Discord, making it a strategic choice. One noteworthy characteristic of JavaScript is its asynchronous capability, allowing developers to handle multiple operations simultaneously.
Advantages of using JavaScript include:
- Seamless integration with Discord.js, a powerful library for building bots.
- A large community and abundant resources for learning.
Disadvantages include:
- Callback hell can make the code less readable if not managed properly.
Python
Python has gained traction in the development community as a favorite for its simplicity and readability. Many developers prefer Python when creating Discord bots, thanks to its clear syntax and extensive libraries. A key feature of Python is its versatility, being effective in both simple and complex tasks.
Advantages of using Python include:
- Ease of learning, making it suitable for beginners.
- Libraries like discord.py simplify the bot-building process.
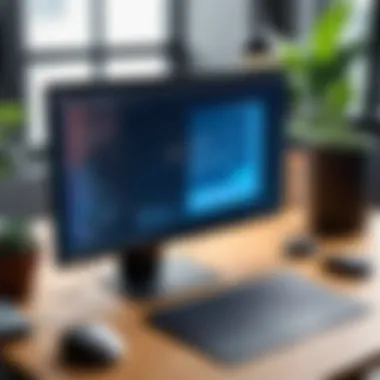
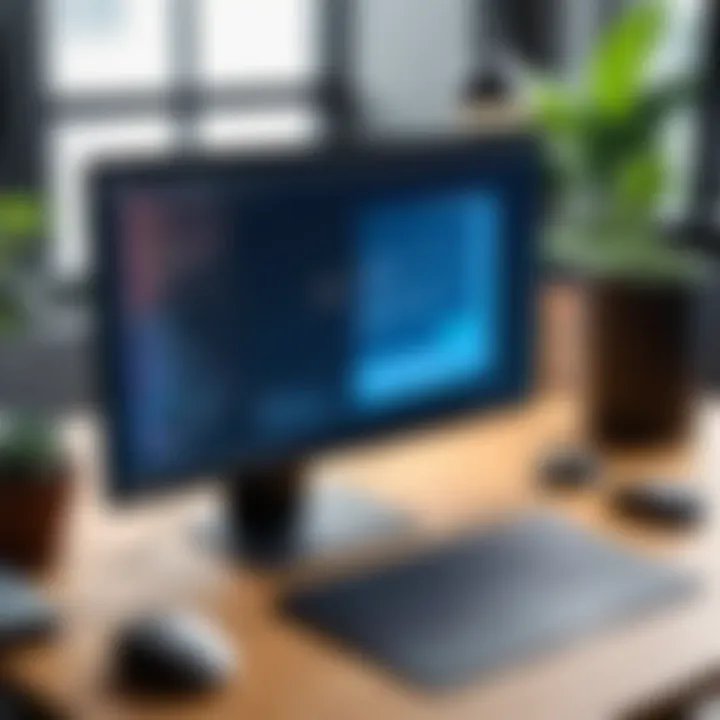
Disadvantages include:
- Slower performance compared to JavaScript, especially for real-time applications.
Java
Java, often seen as a mature and stable option, brings strong typing and extensive libraries to the table. Its platform independence is a significant advantage, allowing bots to run on various operating systems with minimal adjustments. Java's robustness is another reason it's viewed positively among developers.
Advantages of using Java include:
- Strongly typed language helps catch errors early in the development cycle.
- Utilizes a large ecosystem of libraries and frameworks.
Disadvantages include:
- More boilerplate code might be needed, making projects longer to set up.
Installing Required Software
To get started with your Discord bot, installing the right software is crucial. Different languages and tools are required based on the chosen programming language. Below are the necessary installations for JavaScript, Python, and general software recommendations.
Node.js for JavaScript
Node.js is the runtime environment that allows you to run JavaScript outside of a web browser. It is essential for executing JavaScript code on the server-side, making it a core requirement for Discord bot development.
Key features of Node.js include:
- Non-blocking I/O operations make it efficient.
- A rich package repository (npm) that facilitates easy library management.
Advantages:
- Quick prototyping and deployment make it suitable for iterative development.
- The ability to leverage JavaScript for both client and server-side code enhances coherence.
Disadvantages:
- Can be challenging to debug, particularly when dealing with asynchronous code.
Python Libraries
To develop a Discord bot with Python, specific libraries are necessary to facilitate the interaction with Discord's API. Libraries such as discord.py are popular choices that streamline the process.
Key characteristics include:
- Built-in support for most aspects of Discord's API.
- Active community providing updates and support.
Advantages:
- Shorter code for complex tasks due to Python's expressive syntax.
- Good documentation, making it easier for beginners.
Disadvantages:
- Additional libraries may require installation and updates, complicating things a bit.
IDE Recommendations
An Integrated Development Environment (IDE) increases productivity by offering tools for coding, debugging, and deploying your bot. Choosing the right IDE can impact your overall development experience.
Popular IDEs to consider include:
- Visual Studio Code: Highly customizable, supports various languages, including JavaScript and Python.
- PyCharm: A strong option for Python with extensive features for debugging and testing.
- IntelliJ IDEA: Best for Java users, praised for its powerful refactoring capabilities.
Advantages of using an IDE:
- Built-in tools simplify debugging and code completion.
- Many IDEs provide integration with version control systems like Git, enhancing collaboration.
Disadvantages:
- Can be resource-intensive, slowing down lower-spec machines.
In summary, setting up your development environment is an essential step when creating a Discord bot. It involves careful consideration of the programming language and the software you will utilize. A solid foundation will lead to a more efficient development cycle and a better final product.
Creating Your First Bot
Creating your first bot is a crucial step in mastering the functionality offered by Discord's API. This section presents an opportunity to bridge the gap between theory and practice. Engaging with the technicalities of bot creation allows developers to grasp essential concepts that will be necessary for their future projects. This process will solidify your understanding and give you practical skills that can be applied in various other programming scenarios.
Setting Up a Discord Application
To start the process of creating your Discord bot, you must first set up a Discord Application. This is a foundational step that can seem trivial, but it is integral for your bot's functionality. Setting up the application provides the framework through which your bot will operate on Discord. Here are the steps involved:
- Navigate to the Discord Developer Portal: Open your browser and go to Discord's Developer Portal.
- Create a New Application: Click on the “New Application” button. You’ll be prompted to name your application. Choose a name that reflects the purpose of your bot.
- Configure Your Application: Once created, you can configure your application. This includes setting an icon and description. These elements help in identifying your bot on Discord.
- Get Your Application ID: This ID will be essential when integrating your bot with Discord servers. Make sure to keep this ID safe as it’s unique to your application.
The initial application setup is vital as it lays the groundwork for future configurations. Understanding the application structure can also assist when navigating complex features later.
Generating Bot Tokens
After setting up your Discord application, the next step is generating your bot token. A bot token functions as a unique identifier for your bot and a key that allows it to connect to the Discord API. Here's how you can generate and retrieve this token:
- Select Your Application: From the Developer Portal, select the application you created earlier.
- Navigate to the Bot Section: In the application settings, locate the “Bot” tab on the left-hand menu. Click on it.
- Add a Bot: Click on the “Add Bot” button. Discord will ask for confirmation. Accept it to create your bot.
- Retrieve Your Token: After creating the bot, you will see a section labeled “Token.” Click the “Copy” button. Important: Keep this token confidential. If exposed, others may control your bot.
Remember, without this token your bot cannot function. It serves as a direct link between your code and the discord.com infrastructure. Ensure to store it securely, preferably outside your source code, to avoid unauthorized access or potential security breaches.
A bot token should be treated as a password. Never share it publicly or with others.
Coding Your Discord Bot
Understanding how to code your Discord bot is a fundamental step in the development process. This section elaborates on the crucial aspects involved in programming your bot. It outlines not just the mechanics of writing code but also emphasizes the benefits of crafting a well-structured bot for Discord. A well-implemented bot can enhance user experience, automate tasks, and serve specific community needs. Proper coding practices enable maintainability and scalability, which is essential as your bot evolves.
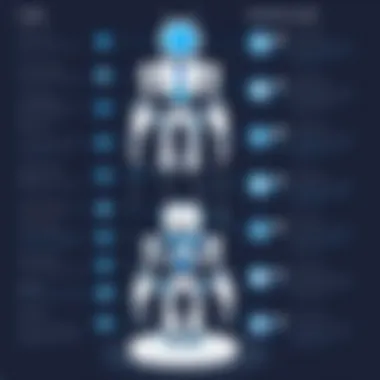
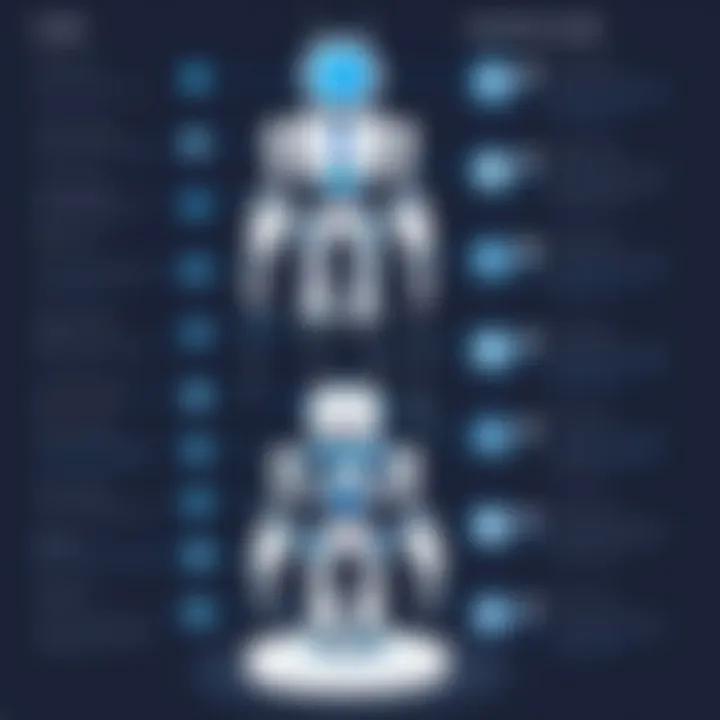
Basic Bot Structure
Every Discord bot has a basic structure that serves as the foundation for its functionality. Initially, you’ll need to set up a simple JavaScript or Python file that will contain the bot’s core code. The primary components of the bot's structure typically include:
- Importing necessary libraries: For example, if you are using the Discord.js library in JavaScript or discord.py in Python, you need to import these libraries to access Discord's API.
- Creating a bot instance: This is where the bot is created and configured with your bot token.
- Logging in: This part of the code enables the bot to connect to Discord using the generated token.
- Event listeners: These listeners facilitate interaction between the bot and Discord’s server, handling events such as messages, user presence, and reactions.
Here is a simplified example of a basic bot structure using JavaScript:
This snippet sets up a basic bot that logs to the console once it is connected. It’s essential to ensure your code is neatly organized to accommodate further functionality.
Implementing Message Commands
Message commands are an integral feature of any Discord bot. They allow users to interact with the bot through simple text inputs. Implementing commands can provide valuable functionality and streamline user engagement. These commands can range from simple responses to complex queries or actions.
Define a command handler in your code. Check for specific prefixes to distinguish commands from regular messages. Use a structure that allows easy addition of new commands later on. Here’s a simple example:
In this case, the bot responds with "Pong!" when a user types "!ping". The command processing can grow in complexity as you integrate more commands, allowing various functionalities.
Handling User Interactions
User interactions are a significant part of a Discord bot's appeal. It is crucial to create code that allows your bot to communicate effectively and respond to user commands in meaningful ways. This includes managing responses to commands and engaging users through reactions, direct messages, and notifications.
To handle interactions, consider the following:
- Reaction commands: Allow users to trigger actions by reacting to messages with emojis.
- Direct messaging: Bots can offer private messaging for sensitive commands or user-specific responses.
- Feedback loops: Consider implementing logs or message acknowledgements that confirm receipt of commands or provide updates on ongoing processes.
An example of handling a reaction command is:
This snippet makes the bot react with a thumbs up emoji when a user sends a specific message.
By structuring your code effectively and considering user interactions, the coding of your Discord bot will yield a responsive and dynamic tool for your community.
Testing and Debugging
Testing and debugging are crucial stages in the development of a Discord bot. These processes ensure that the bot operates as intended, responding accurately to user commands and maintaining a stable performance. A well-tested bot enhances user experience. It minimizes errors and unexpected behavior, which can frustrate users and degrade trust in the bot's capabilities.
When a developer tests a bot, they are often looking for specific issues and bugs that may arise during normal operation. Debugging tools can help identify the source of errors quickly, allowing for more efficient corrections. In addition, routine maintenance such as regular debugging contributes to the long-term effectiveness of the bot since it allows developers to update the bot for performance and security.
Debugging Tools and Techniques
There are several tools and techniques that developers can utilize to debug their Discord bots effectively. These include:
- Console Logging: The simplest debugging method is to use console.log statements to track the flow of the bot's behavior. Logging relevant information can help pinpoint when an error occurs but developers should be cautious not to overload the console with excessive information.
- Node.js Debugger: For JavaScript-based bots, the Node.js Debugger allows developers to set breakpoints and inspect variable states during execution. This method provides deeper insight into the bot's functionality.
- Visual Studio Code Debugger: Developers using Visual Studio Code can take advantage of its built-in debugging features. It offers a user-friendly interface for setting breakpoints and stepping through code.
- Error Tracking Services: Tools like Sentry or Rollbar can be integrated to help capture runtime errors and monitor performance in real time. They provide a comprehensive overview of the bot’s performance and any issues that arise.
Employing these tools can streamline the debugging process, making it more efficient and effective.
Conducting Functional Tests
Functional testing involves systematically evaluating the bot to ensure all features work as intended. This process typically includes:
- Unit Testing: Isolate and test individual functions or components of the bot to verify their correctness. Many frameworks, like Jest for JavaScript or Pytest for Python, aid in creating unit tests.
- Integration Testing: Assess how various components interact with each other within the bot. This is important because interactions can lead to unforeseen issues that unit tests may not catch.
- User Acceptance Testing (UAT): Share the bot with a group of typical users to gather feedback on its functionality. This feedback helps identify any usability issues or bugs that were not apparent during earlier testing phases.
- Performance Testing: Evaluate the bot under different loads to ensure it can handle multiple users and commands without degradation in performance.
When conducting functional tests, it is vital to document all findings meticulously to facilitate easier debugging later. This allows developers to revisit tests and improve their bots accordingly.
Deploying Your Discord Bot
Deploying your Discord bot is a crucial phase in the development process. Once you have written the code and tested the functionality, it is time to ensure that it runs in a reliable environment. This step influences the availability, performance, and scalability of your bot. A poorly deployed bot may lead to downtime, missed interactions, and a negative user experience. Therefore, seeing through the various deployment options and their implications is essential.
Choosing a Hosting Solution
Choosing the right hosting solution is fundamental for your Discord bot's success. Each option provides varying levels of performance, control, and cost-effectiveness. The three main types of hosting solutions are mentioned below.
Cloud Hosting
Cloud hosting is a modern solution that offers scalability and flexibility. It allows your bot to leverage resources from networked servers rather than relying on a single machine. One key characteristic is the pay-as-you-go model, meaning you only pay for what you use. This model makes it a popular choice for developers who want to minimize upfront costs.
A unique feature of cloud hosting is its ability to automatically adjust to varying traffic loads. If your bot experiences sudden spikes in user activity, cloud hosting can allocate more resources without manual intervention. The major advantage of cloud hosting is its high availability and ability to scale quickly. However, potential downsides include variable costs which can escalate unexpectedly during high usage periods.
Dedicated Servers
For developers seeking more control, dedicated servers present a viable option. A dedicated server grants you an entire physical server for your bot. This exclusivity ensures optimal performance without interference from other applications. One key characteristic is the predictable pricing model, where you pay a fixed monthly fee regardless of usage.
The unique feature of dedicated servers is the control over hardware and operating systems. This allows for specific configurations optimized for your bot. However, managing a dedicated server requires technical knowledge, which may not be ideal for all developers. The potential disadvantages include higher costs and responsibilities for maintenance and security oversight.
Free Hosting Options
For those with tight budgets or just starting, free hosting options can be appealing. Many platforms offer limited hosting plans at no cost. The key characteristic of free hosting is the absence of financial barriers, enabling new developers to try their hands at building bots without significant investment.
Unique features often include simple setup procedures and community support. While this might seem beneficial, there are drawbacks. Free hosting solutions may impose strict limitations on resources, such as bandwidth or uptime. Moreover, reliability can be compromised, leaving your bot vulnerable to unplanned downtime.
Starting the Bot on a Server
Once you have chosen a hosting solution, the final step is to start your bot on a server. This process involves deploying the code and ensuring all dependencies are properly set. Each hosting solution may have different procedures, but the general steps remain similar. You'll need to configure the environment to match what the bot requires to function effectively.
Maintaining and Updating Your Bot
Maintaining and updating your Discord bot is a critical aspect that cannot be overlooked. After you have deployed your bot, its performance, functionality, and user engagement will continually evolve. Regular maintenance ensures that your bot operates smoothly and meets the needs of your users effectively. Ignoring these responsibilities can lead to bugs, outdated features, and user frustration. It is also vital for keeping security threats in check. Disregarding updates can leave your bot vulnerable to exploits and malware, putting both your server and users at risk.
Monitoring Bot Performance
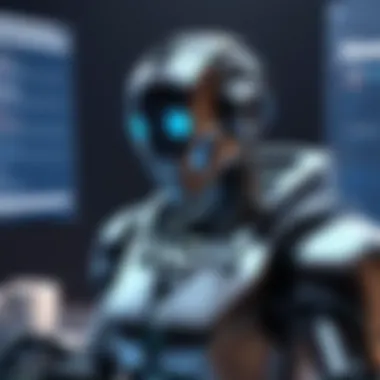
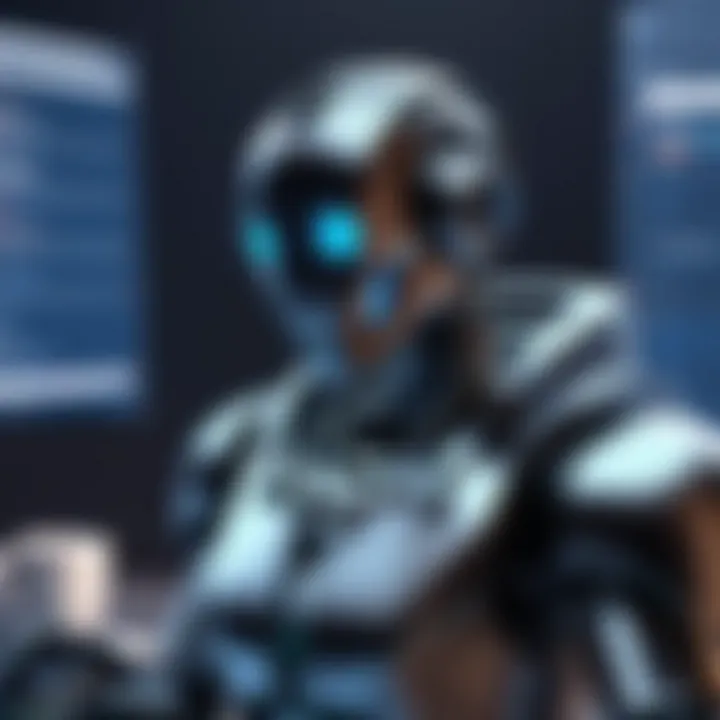
Monitoring your bot’s performance is essential to understand how it functions in a real-world environment. Metrics such as response time, uptime, and error rates provide insight into the bot's reliability. A few strategies to monitor performance include:
- Logging and Analytics: Implement logging to track errors and interactions. Use a service like Sentry to catch issues before they affect users completely.
- User Engagement Metrics: Monitor how often users interact with your bot. High interaction rates can indicate usefulness, while low rates might suggest that there are issues to address.
- Performance Testing: Regularly conduct load tests to ensure that your bot can handle increased user activity. Consider tools like Artillery or Apache JMeter for stress testing.
By setting up a robust monitoring system, you can make data-driven decisions about when and how to update your bot, ensuring that it remains user-friendly and responsive.
Implementing User Feedback
User feedback is a goldmine for improving your bot. It offers direct insights from those who actually use your service. Consider these methods to gather and implement feedback:
- Surveys and Polls: Use platforms like Google Forms or SurveyMonkey to run surveys among your user base. Ask specific questions about what features they like and what could be improved.
- Engagement in Chats: Encourage users to voice their opinions directly in your Discord server. Create a dedicated channel for feature suggestions or bug reports.
- Regular Updates: Keep users informed about what changes you are making based on feedback. This transparency can strengthen community ties.
Understanding user preferences helps tailor your bot to meet expectations effectively, increasing user satisfaction. Moreover, it reinforces the idea that you value and respond to user input, fostering a stronger relationship with your user base.
"User feedback is the cornerstone of a successful product. It is through listening and adjusting that we create real value."
By integrating performance monitoring and user feedback into your maintenance strategy, you can ensure your Discord bot not only meets current expectations but also adapts to future needs.
Exploring Advanced Features
Incorporating advanced features into your Discord bot enhances its functionality and user experience. These features can significantly differentiate your bot from others in the crowded Discord ecosystem. Adding sophistication to your bot can involve complex commands, integrations, or simply improving interaction quality. This section will delve into two notable advanced features: music capabilities and reaction roles.
Adding Music Capabilities
Music features in a Discord bot elevate the entertainment aspect for users. Users appreciate a bot that can play music from various sources. This feature invites greater engagement during server gatherings or events. To implement this ability, you can use libraries such as or , which provide the necessary functions for streaming audio.
Getting started typically involves several key steps:
- Selecting a Source: Decide whether your bot will pull music from platforms like YouTube, Spotify, or SoundCloud. Each platform may have varying levels of API accessibility.
- Using Libraries: Integrate libraries specialized for audio streaming. For example, has a built-in package that simplifies voice connection and playback.
- Creating Commands: The bot should recognize commands like or . Implementing an asynchronous processing method for handling user commands is crucial.
Here’s a simple code snippet demonstrating music playback functionality:
Considerations: Implementing music capabilities may require navigating copyright issues. It is essential to ensure compliance with each music platform's terms of service. This diligence will prevent potential legal risks.
Implementing Reaction Roles
Reaction roles provide a dynamic way for users to select their roles within your Discord server. This feature simplifies role allocation and increases user autonomy, encouraging interaction. When a user reacts to a designated message with specific emojis, the bot assigns them roles accordingly.
To implement reaction roles, consider the steps outlined below:
- Message Setup: First, send a message that describes available roles using specific emojis. Users need clear instructions.
- Listening for Reactions: The bot must listen for reactions on the designated message. Implement appropriate event listeners to capture when users add or remove their reactions.
- Assigning and Removing Roles: Based on the emoji selected, the bot should assign the corresponding role to the user, or remove it when they unreact.
Here is an example code illustrating how to set up reaction roles:
Benefits: Reaction roles enhance server organization and personalization. Users feel more engaged and empowered. Moreover, establishing clearer role structures leads to better community management.
Through the integration of advanced features like music capabilities and reaction roles, the functionality of your Discord bot can significantly improve. This not only attracts users but also fosters an active and thriving community.
Security Considerations
Security is a fundamental aspect when developing a Discord bot. As bots interact with users and manage data, they become potential targets for malicious actors. Understanding security considerations helps developers protect their bot and the community using it. Failing to implement adequate security measures can lead to unauthorized access, data breaches, and misuse of the bot's functionalities. Thus, prioritizing security ensures that bot operations run smoothly and safely, fostering a reliable user experience.
Protecting Your Bot Token
The bot token functions like a password, granting access to the bot's capabilities through the Discord API. It is crucial to safeguard this token from any unauthorized exposure. If someone gains access to your bot token, they can impersonate your bot and possibly cause harm.
Here are key practices to protect the bot token:
- Do not hard-code the token in your source code. Instead, use environment variables or configuration files that are not included in public repositories.
- Limit access. Only share the bot token with trusted individuals who need it for development or management purposes.
- Regenerate the token if it's exposed. Discord allows you to create a new token in the developer portal, which invalidates the old one.
By following these best practices, you can minimize the risk of compromising your bot's security.
Handling Spam and Abuse
As bots can interact with numerous users in a Discord server, they are susceptible to spam and abuse. Malicious users may exploit bots to send unsolicited messages or engage in disruptive behavior. Therefore, it is vital to have mechanisms in place to handle such situations effectively.
Consider the following strategies for managing spam and abuse:
- Implement rate limits. This restricts the number of commands a user can invoke within a specific time frame. Establishing a buffer helps prevent abuse while allowing legitimate interactions.
- Set up user verification methods. Requiring new users to verify their identity before accessing certain bot functionalities can reduce spam activities.
- Monitor and ban abusive users. Create functions to track user behavior and automatically ban or mute users who violate community guidelines.
By actively implementing these strategies, you can create a more secure and enjoyable environment for users and mitigate the risk of spam and abuse.
Community and Support
Creating a Discord bot involves more than just coding. It requires an understanding of the community surrounding Discord and the support mechanisms available to developers. Establishing connections with fellow developers can enhance the quality of your bot and facilitate easier problem-solving. Community support provides not only guidance but also encouragement. Developers can share their experiences, recommend tools, and suggest best practices, which can lead to a more effective design and implementation of your bot.
Engaging with a community allows developers to stay updated on trends and changes that might affect their bot’s performance or security. This is particularly important in a fast-paced environment like Discord, where APIs and features may frequently change.
Finding Helpful Resources
When creating a bot, locating reliable resources is essential. Resources can range from official documentation to tutorials posted by experienced developers. Here are some key places to look:
- Official Discord Documentation: The official Discord Developer Portal offers comprehensive and up-to-date information on bot development, the Discord API, and other features.
- Online Forums: Websites like Reddit and Stack Overflow are valuable platforms for asking specific questions and getting answers from fellow developers.
- YouTube Tutorials: Video tutorials can be especially helpful for visual learners. Many experienced developers share detailed guides on YouTube, covering various aspects of bot development.
Utilizing these resources not only speeds up the learning process but also provides you with insights that may not be easily accessible through official channels.
Joining Developer Communities
Connecting with other developers who share your interest in bot creation can be beneficial. Online communities provide a space for brainstorming, troubleshooting, and collaboration. Several communities are specifically geared towards Discord developers, such as:
- Discord Servers: Many developers have created Discord servers dedicated to bot development. These are real-time, interactive environments where you can ask for help and share ideas.
- Facebook Groups: There are various groups on Facebook where developers exchange knowledge and discuss challenges they face during bot creation.
- Meetups and Conferences: Attending local meetups or technology conferences can provide face-to-face networking opportunities with other developers.
Engaging in these communities offers not only technical support but also encourages creativity through collaboration. Surrounding yourself with other passionate developers can inspire new ideas for your bot and keep you motivated in your development journey.
"Community is a major key to valuable growth for any developer. Building relationships can change everything."
By actively participating in communities and utilizing available resources, developers can create high-quality Discord bots that attract and retain users. Balancing technical skills with community engagement often leads to successful projects that stand out in a competitive arena.